Overview
The Indexceptional API enables programmatic indexing request management. You can check credit balances, submit URLs for indexing, and monitor order statuses.
Authentication
The API uses Basic Authentication with WordPress Application Passwords. To get started:
- Log in to your Indexceptional account at https://www.indexceptional.com
- Go to your Account page https://www.indexceptional.com/account
- Select API Access Tab
- Create a new application password for “API Access”
- Use your username and the generated password for API requests
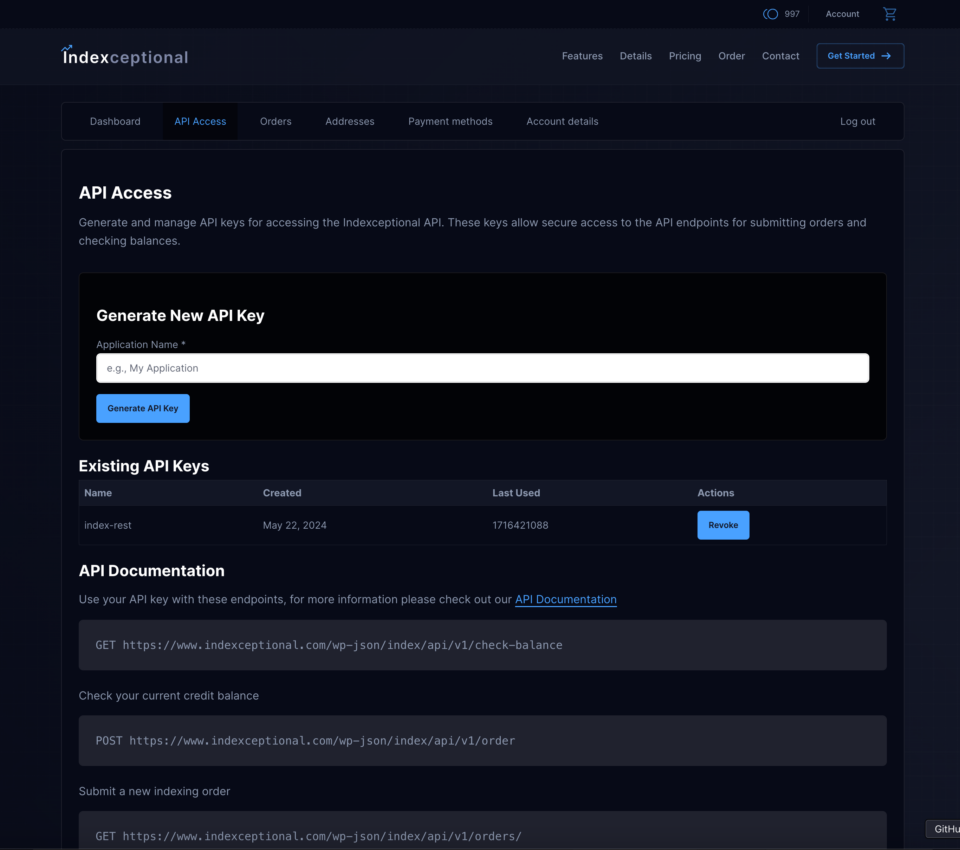
Base URL
https://www.indexceptional.com/wp-json/index/api/v1
URL Components
The base URL consists of several components:
- Domain:
https://www.indexceptional.com
- Production environment
- Secure HTTPS connection required
- www subdomain required
- WordPress REST API prefix:
/wp-json
- Standard WordPress REST API endpoint
- Cannot be modified
- Required for all API requests
- API Namespace:
/index/api
- Unique to Indexceptional
- Separates our endpoints from other WordPress APIs
- Version independent
- Version:
/v1
- Current API version
- Major version changes may break compatibility
- Used for API versioning and backwards compatibility
HTTP Protocols
- HTTPS is required for all API requests
- HTTP requests will be automatically redirected to HTTPS
- SSL/TLS certificates must be valid
- Minimum TLS version: 1.2
Request Requirements
Headers
Authorization: Basic {credentials}
Content-Type: application/json
Accept: application/json
Rate Limiting Headers
X-RateLimit-Limit: {requests_per_hour}
X-RateLimit-Remaining: {remaining_requests}
X-RateLimit-Reset: {timestamp}
URL Parameters
When adding query parameters to endpoints that support them:
https://www.indexceptional.com/wp-json/index/api/v1/orders?per_page=20&page=1&status=completed
Parameters must be URL encoded Multiple parameters joined with & Boolean values should be ‘true’ or ‘false’ Arrays can be specified with [] notation
Endpoints
Check Balance
Retrieves the current credit balance for the authenticated user.
https://www.indexceptional.com/wp-json/index/api/v1/check-balance
Endpoint: /check-balance
Method: GET
Authentication Required: Yes
This endpoint returns the user’s current credit balance and indicates if they have unlimited credits. It’s useful for checking if you have sufficient credits before submitting an order.
Parameters
None required
Response Fields
credits
(integer): Current number of credits availableunlimited
(boolean): Whether the user has unlimited credits
Example Response
{
"success": true,
"data": {
"credits": 100,
"unlimited": false
}
}
Common Error Codes
rest_not_logged_in
: User is not authenticatedrest_no_route
: Invalid endpoint URL
Code Examples
cURL
PHP
Node JS
Python
Submit Order
Submit URLs for indexing.
https://www.indexceptional.com/wp-json/index/api/v1/order
Endpoint: /order
Method: POST
Authentication Required: Yes
This endpoint allows you to submit URLs for indexing. Each URL submitted will use one credit from your balance. The order will be processed according to our indexing queue system.
Rate Limits
- Maximum 500 URLs per order
- Minimum 20 seconds between requests
- Maximum 10 orders per day per user
Request Parameters
Parameter | Type | Required | Description |
---|---|---|---|
urls | array | Yes | Array of URLs to be indexed |
Request Body Example
{
"urls": [
"https://example.com/page1",
"https://example.com/page2"
]
}
Response Fields
order_id
(integer): Unique identifier for the orderstatus
(string): Current status of the ordertotal_urls
(integer): Number of URLs in the orderurls
(array): Array of URL objects with their status
Example Response
{
"success": true,
"data": {
"order_id": 12345,
"status": "api-ordered",
"total_urls": 2,
"urls": [
{
"url": "https://example.com/page1",
"date_created": "2025-02-06",
"status": "not-started"
},
{
"url": "https://example.com/page2",
"date_created": "2025-02-06",
"status": "not-started"
}
]
}
}
Common Error Codes
too_many_urls
: Exceeded maximum URLs per orderrate_limit_exceeded
: Request made too soon after previous requestdaily_limit_exceeded
: Exceeded maximum orders per dayinsufficient_credits
: Not enough credits for the order
Code Examples
cURL
PHP
Node JS
Python
Get Orders Numbers
Retrieve a list of your orders.
https://www.indexceptional.com/wp-json/index/api/v1/orders
Endpoint: /orders
Method: GET
Authentication Required: Yes
This endpoint returns a paginated list of your orders. You can filter orders by status and control the number of results per page.
Query Parameters
Parameter | Type | Required | Default | Description |
---|---|---|---|---|
per_page | integer | No | 10 | Number of orders per page |
page | integer | No | 1 | Page number to retrieve |
status | string | No | ‘any’ | Filter by order status |
Available Status Values
any
: All statusesapi-ordered
: Initial order statemanual-action
: Requires manual interventiongbot-visited
: Google bot has visitedchecking-index
: Checking index statuscompleted
: Indexing completed
Response Fields
orders
(array): Array of order IDstotal
(integer): Total number of ordersper_page
(integer): Orders per pagecurrent_page
(integer): Current page numbertotal_pages
(integer): Total number of pages
Example Response
{
"success": true,
"data": {
"orders": [12345, 12346, 12347],
"total": 50,
"per_page": 10,
"current_page": 1,
"total_pages": 5
}
}
Common Error Codes
invalid_status
: Invalid status filter providedinvalid_page
: Page number out of range
Code Examples
cURL
PHP
Node JS
Python
Check Order Status
Get the status of a specific order.
https://www.indexceptional.com/wp-json/index/api/v1/order/status/{order_id}
Endpoint: /order/status/{order_id}
Method: GET
Authentication Required: Yes
This endpoint allows you to check the current status of an existing order. It returns detailed information about each URL in the order and its indexing progress.
URL Parameters
Parameter | Type | Required | Description |
---|---|---|---|
order_id | integer | Yes | ID of the order to check |
Response Fields
order_id
(integer): ID of the orderstatus
(string): Overall order statusdate_created
(string): Order creation datetotal_urls
(integer): Total URLs in the orderurls
(array): Array of URL objects with detailed status
Example Response
{
"success": true,
"data": {
"order_id": 12345,
"status": "processing",
"date_created": "2025-02-06T12:00:00",
"total_urls": 2,
"urls": [
{
"url": "https://example.com/page1",
"indexable": "200",
"date_created": "2025-02-06",
"status": "gbot-visited",
"date_indexed": "2025-02-06",
"serp": "Indexed successfully"
},
{
"url": "https://example.com/page2",
"indexable": "200",
"date_created": "2025-02-06",
"status": "checking-index",
"date_indexed": "-",
"serp": "-"
}
]
}
}
Common Error Codes
order_not_found
: Order ID doesn’t existunauthorized
: Not authorized to view this order
Code Example
cURL
PHP
Node JS
Python
Response Formats
Success Response
{
"success": true,
"data": {
// Response data varies by endpoint
}
}
Error Response
{
"code": "error_code",
"message": "Error message description",
"data": {
"status": 400
}
}
API Restrictions
The following restrictions apply to order submissions:
- URL Limit
- Maximum 500 URLs per order
- URLs must be valid and accessible
- Each URL counts as one credit
- Request Timing
- Minimum 20 seconds between requests
- Rate limit applies per user account
- Exceeded limits return a 429 status code
- Daily Order Limit
- Maximum 10 orders per day
- Resets at midnight UTC
- Limit applies per user account
Error Responses
Too Many URLs
{
"code": "too_many_urls",
"message": "Maximum of 500 URLs allowed per order. You provided 750 URLs.",
"data": {
"status": 400
}
}
Rate Limit Exceeded
{
"code": "rate_limit_exceeded",
"message": "Please wait 15 seconds before submitting another request.",
"data": {
"status": 429
}
}
Daily Limit Exceeded
{
"code": "daily_limit_exceeded",
"message": "Maximum of 10 orders per day reached. Please try again tomorrow.",
"data": {
"status": 429
}
}
Status Code
Status Code | Description |
---|---|
200 | Success |
400 | Bad Request |
401 | Unauthorized |
403 | Forbidden |
404 | Not Found |
429 | Too Many Requests |
500 | Server Error |
Order Status Flow
URLs in orders follow this status progression:
not-started
→ Initial stategbot-visited
→ Google bot has visitedchecking-index
→ Verifying index statuscompleted
→ Successfully indexed
Each status update is reflected in the order status endpoint response.
Best Practices
- Implement retry logic with exponential backoff
- Monitor response headers for rate limit information
- Batch URLs efficiently within the 500 URL limit
- Track daily order count to avoid hitting limits
- Handle rate limit errors gracefully in your application
Need Help?
For support or questions, contact us at:
- Email: [email protected]
- Website: https://www.indexceptional.com/support